At regular intervals, the Open Web Application Security Project (OWASP) positions the most basic web application security chances. Starting from the primary report, infusion gambles have forever been on top. Among all infusion types, SQL infusion is quite possibly the most widely recognized assault vector, and apparently the most perilous. As Python is one of the most famous programming dialects on the planet, knowing how to safeguard against Python SQL injection is basic.
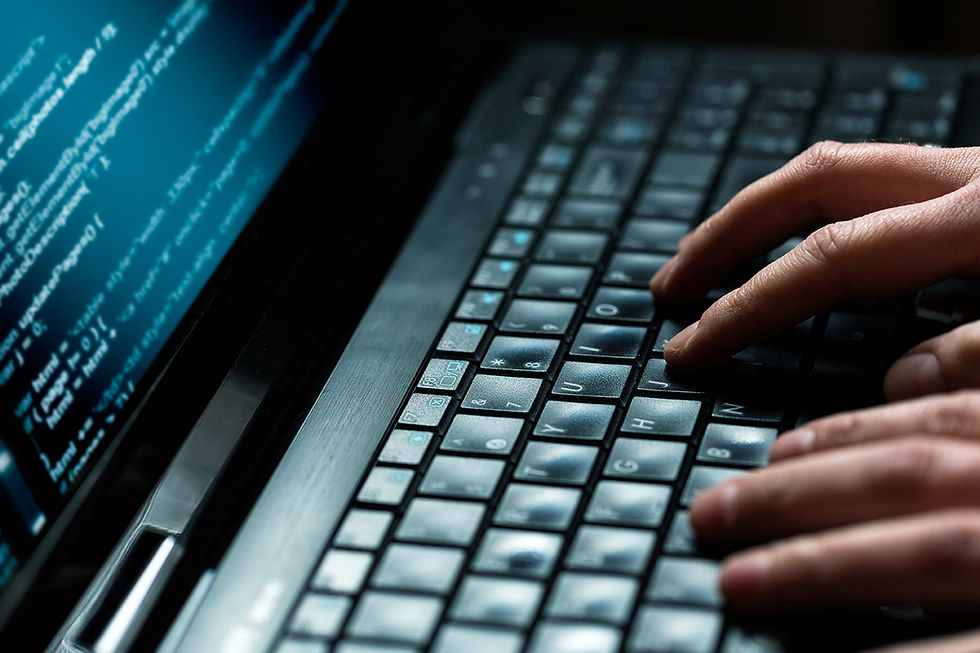
In this instructional exercise, you will learn:
What Python SQL infusion is and how to forestall it
Instructions to create questions with the two literals and identifiers as boundaries
The most effective method to securely execute questions in a data set
This instructional exercise is appropriate for clients of all information base motors. The models here use PostgreSQL injection, however the outcomes can be repeated in other data set administration frameworks (like SQLite, MySQL, Microsoft SQL Server, Oracle, etc).
READ NOW - Python for beginners
Free Bonus: 5 Thoughts On Python Mastery, a free course for Python designers that shows you the guide and the mentality you'll have to take your Python abilities to a higher level.
Figuring out Python SQL Injection
SQL Injection assaults are such a typical security weakness that the unbelievable xkcd webcomic gave a comic to it:
A silly webcomic by xkcd about the likely impact of SQL infusion
"Exploits of a Mom" (Image: xkcd)
Producing and executing SQL inquiries is a typical errand. In any case, organizations all over the planet frequently commit horrendous errors with regards to creating SQL articulations. While the ORM layer ordinarily creates SQL questions, at times you need to compose your own.
At the point when you use Python to execute these inquiries straightforwardly into an information base, there's an opportunity you could commit errors that could think twice about framework. In this instructional exercise, you'll figure out how to effectively execute capacities that make dynamic SQL questions without endangering your framework for Python SQL infusion.
Eliminate promotions
Setting Up a Database
To begin, you will set up a new PostgreSQL data set and populate it with information. All through the instructional exercise, you'll utilize this data set to observe firsthand how Python SQL infusion functions.
Making a Database
To begin with, open your shell and make another PostgreSQL data set possessed by the client postgres:
$ createdb - O postgres psycopgtest
Here you utilized the order line choice - O to set the proprietor of the data set to the client postgres. You likewise determined the name of the information base, which is psycopgtest.
READ NOW - Python for beginners
Note: postgres is an extraordinary client, which you would ordinarily save for regulatory undertakings, however for this instructional exercise, it's fine to utilize postgres. In a genuine framework, in any case, you ought to make a different client to be the proprietor of the data set.
Your new information base is all set! You can associate with it utilizing psql:
$ psql - U postgres - d psycopgtest
psql (11.2, server 10.5)
Type "help" for help.
You're currently associated with the information base psycopgtest as the client postgres. This client is additionally the data set proprietor, so you'll have perused authorizations on each table in the data set.
Making a Table With Data
Then, you want to make a table with a client data and add information to it:
psycopgtest=# CREATE TABLE clients (
username varchar(30),
administrator boolean
);
Make TABLE
psycopgtest=# INSERT INTO clients
(username, administrator)
VALUES
('ran', valid),
('haki', misleading);
Embed 0 2
psycopgtest=# SELECT * FROM clients;
username | administrator
----------+-------
ran | t
haki | f
(2 columns)
The table has two segments: username and administrator. The administrator segment demonstrates whether a client has managerial honors. You want to focus on the administrator field and attempt to mishandle it.
Setting Up a Python Virtual Environment
Since you have an information base, now is the right time to set up your Python climate. For bit by bit guidelines on the most proficient method to do this, look at Python Virtual Environments: A Primer.
Establish your virtual climate in another registry:
(~/src) $ mkdir psycopgtest
(~/src) $ album psycopgtest
(~/src/psycopgtest) $ python3 - m venv
After you run this order, another registry called venv will be made. This catalog will store every one of the bundles you introduce inside the virtual climate.
Associating with the Database
To associate with a data set in Python, you really want a data set connector. Most information base connectors follow adaptation 2.0 of the Python Database API Specification PEP 249. Each significant data set motor has a main connector:
Database Adapter
PostgreSQL Psycopg
SQLite sqlite3
Oracle cx_oracle
MySql MySQLdb
To interface with a PostgreSQL data set, you'll have to introduce Psycopg, which is the most famous connector for Postgre SQL injection in Python. Django ORM utilizes it naturally, and it's additionally upheld by SQLAlchemy.
In your terminal, actuate the virtual climate and use pip to introduce psycopg:
(~/src/psycopgtest) $ source venv/receptacle/initiate
(~/src/psycopgtest) $ python - m pip introduce psycopg2>=2.8.0
Gathering psycopg2
Utilizing stored https://....
psycopg2-2.8.2.tar.gz
Introducing gathered bundles: psycopg2
Running setup.py introduce for psycopg2 ... done
Effectively introduced psycopg2-2.8.2
Presently you're prepared to make an association with your information base. Here is the beginning of your Python script:
import psycopg2
association = psycopg2.connect(
host="localhost",
database="psycopgtest",
user="postgres",
password=None,
)
connection.set_session(autocommit=True)
You utilized psycopg2.connect() to make the association. This capacity acknowledges the accompanying contentions:
have is the IP address or the DNS of the server where your information base is found. For this situation, the host is your nearby machine, or localhost.
information base is the name of the data set to interface with. You need to associate with the data set you made before, psycopgtest.
client is a client with consents for the information base. For this situation, you need to interface with the information base as the proprietor, so you pass the client postgres.
secret key is the secret phrase for whoever you determined in client. In most improvement conditions, clients can associate with the nearby data set without a secret word.
In the wake of setting up the association, you designed the meeting with autocommit=True. Enacting autocommit implies you will not need to physically oversee exchanges by giving a commit or rollback. This is the default conduct in many ORMs. You utilize this conduct here also so you can zero in on making SQL questions rather out of overseeing exchanges.
Note: Django clients can get the case of the association utilized by the ORM from django.db.connection:
from django.db import association
Eliminate advertisements
Executing a Query
Since you have an association with the data set, you're prepared to execute an inquiry:
>>> with connection.cursor() as cursor:
... cursor.execute('SELECT COUNT(*) FROM clients')
... result = cursor.fetchone()
... print(result)
(2,)
You utilized the association object to make a cursor. Very much like a record in Python, cursor is carried out as a setting director. At the point when you make the specific circumstance, a cursor is opened for you to use to send orders to the data set. At the point when the setting exits, the cursor closes and you can never again utilize it.
Note: To study setting directors, look at Python Context Managers and the "with" Statement.
While inside the unique circumstance, you utilized cursor to execute a question and bring the outcomes. For this situation, you gave a question to include the lines in the clients table. To bring the outcome from the question, you executed cursor.fetchone() and got a tuple. Since the question can return one outcome, you utilized fetchone(). In the event that the question were to return more than one outcome, you'd have to either repeat over cursor or utilize one of the other fetch* techniques.
Involving Query Parameters in SQL injection
In the past segment, you made a data set, laid out an association with it, and executed a question. The question you utilized was static. All in all, it had no boundaries. Presently you'll begin to involve boundaries in your questions.
To start with, you will execute a capacity that checks whether a client is an administrator. is_admin() acknowledges a username and returns that client's administrator status:
Eliminate promotions
Taking advantage of Query Parameters With Python SQL Injection
In the past model, you utilized string addition to produce a question. Then, you executed the inquiry and sent the subsequent string straightforwardly to the information base. Be that as it may, there's something you might have neglected during this interaction.
TRENDING - Python for beginners
Recall the username contention you passed to is_admin(). What precisely does this variable address? You could expect that username is only a string that addresses a real client's name. As you're going to see, however, a gatecrasher can without much of a stretch endeavor this sort of oversight and really hurt major by performing Python SQL infusion.
>>> print("select administrator from clients where username = '%s'" % "'; select valid; - - ")
select administrator from clients where username = ''; select valid; - - '
The subsequent text contains three proclamations. To see precisely how Python SQL infusion functions, you want to examine each part independently. The principal articulation is as per the following:
--'
This scrap stops anything that comes after it. The gatecrasher added the remark image (- - ) to turn all that you could have put after the last placeholder into a remark.
At the point when you execute the capacity with this contention, it will constantly bring True back. If, for instance, you utilize this capacity in your login page, a gatecrasher could sign in with the username '; select valid; - - , and they'll be conceded admittance.
Comments