Face Recognition with Python
- papaki laou
- May 24, 2022
- 5 min read
In this article, we'll take a gander at a shockingly basic method for beginning with face acknowledgment utilizing Python and the open source library OpenCV.
Before you pose any inquiries in the remarks segment:
Try not to avoid the article and simply attempt to run the code. You should comprehend what the code does, not exclusively to run it appropriately yet additionally to investigate it.
Make a point to utilize OpenCV v2.
Have a functioning webcam so this content can work appropriately.
Survey different remarks and questions, since your inquiries have likely as of now been tended to.
Much thanks to you.
LEARN TODAY - Learn bash scripting
Free Bonus: Click here to get the Python Face Detection and OpenCV Examples Mini-Guide that shows you pragmatic code instances of certifiable Python PC vision strategies.
Note: Also look at our refreshed instructional exercise on face identification utilizing Python.
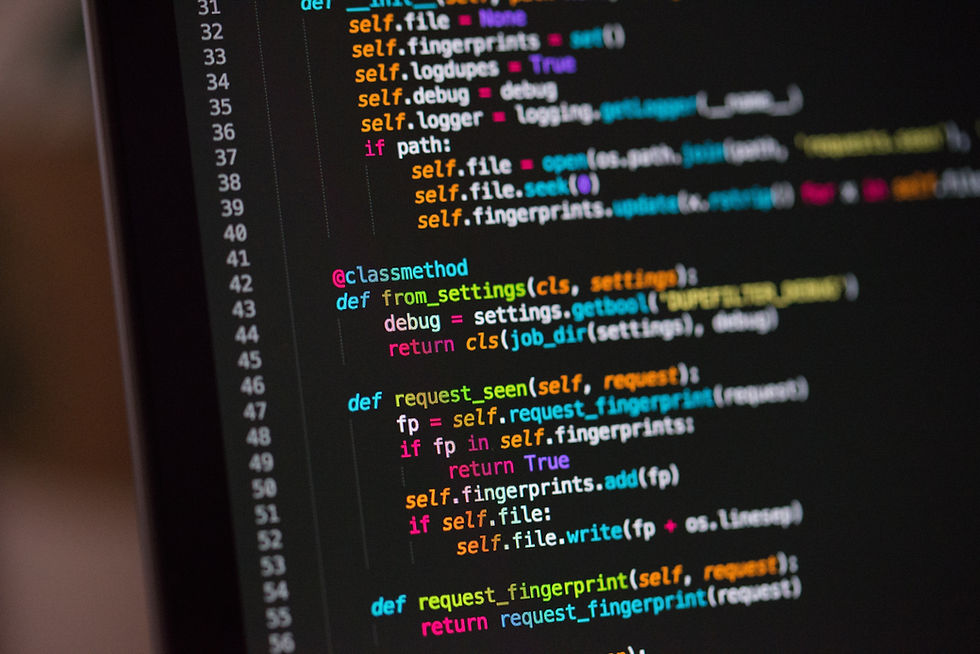
OpenCV
OpenCV is the most famous library for PC vision. Initially written in C/C++, it presently gives ties to Python.
OpenCV utilizes AI calculations to look for faces inside an image. Since faces are so convoluted, there isn't one straightforward test that will let you know if it tracked down a face or not. All things being equal, there are large number of little examples and elements that should be coordinated. The calculations break the errand of recognizing the face into huge number of more modest, scaled down undertakings, every one of which is not difficult to settle. These errands are likewise called classifiers.
TODAY - Learn bash scripting
For something like a face, you could have at least 6,000 classifiers, all of which should match for a face to be recognized (inside mistake limits, obviously). Yet, in that lies the issue: for face discovery, the calculation begins at the upper left of an image and gets down across little blocks of information, taking a gander at each block, continually inquiring, "Is this a face? … Is this a face? … Is this a face?" Since there are at least 6,000 tests for every block, you could have a huge number of computations to do, which will crush your PC to a stop.
To get around this, OpenCV utilizes overflows. What's an outpouring? The most fitting response can be tracked down in the word reference: "a cascade or series of cascades."
Like a progression of cascades, the OpenCV overflow breaks the issue of identifying faces into various stages. For each block, it does an extremely harsh and fast test. Assuming that that passes, it does a somewhat more definite test, etc. The calculation might have 30 to 50 of these stages or fountains, and it will possibly distinguish a face in the event that all stages pass.
The benefit is that most of the image will return a negative during the initial not many stages, and that implies the calculation won't sit around idly testing each of the 6,000 elements on it. Rather than requiring hours, face recognition should this present time be possible in genuine opportunity.
Eliminate advertisements
Overflows in Practice
However the hypothesis might sound muddled, practically speaking it is very simple. The actual fountains are only a lot of XML records that contain OpenCV information used to distinguish objects. You introduce your code with the outpouring you need, and afterward it accomplishes the work for you.
Since face discovery is a particularly normal case, OpenCV accompanies various underlying fountains for recognizing all that from countenances to eyes to hands to legs. There are even fountains for non-human things. For instance, if you run a banana shop and need to follow individuals taking bananas, this person has fabricated one for that!
Introducing OpenCV
In the first place, you want to find the right arrangement document for your working framework.
I observed that introducing OpenCV was the hardest piece of the undertaking. On the off chance that you get weird unexplainable mistakes, it very well may be because of library conflicts, 32/64 cycle contrasts, etc. I found it simplest to utilize a Linux virtual machine and introduce OpenCV without any preparation.
Whenever you have finished the establishment, you can test whether it works by starting up a Python meeting and composing:
>>> import cv2
>>>
On the off chance that you get no mistakes, you can continue on toward the following part.
Grasping the Code
How about we figure out down the genuine code, which you can download from the repo. Snatch the face_detect.py script, the abba.png pic, and the haarcascade_frontalface_default.xml.
# Get client provided values
imagePath = sys.argv[1]
cascPath = sys.argv[2]
You first pass in the picture and fountain names as order line contentions. We'll utilize the ABBA picture as well as the default overflow for recognizing faces given by OpenCV.
# Make the haar overflow
faceCascade = cv2.CascadeClassifier(cascPath)
Presently we make the outpouring and instate it with our face overflow. This heaps the face overflow into memory so it's prepared for use. Keep in mind, the outpouring is only a XML document that contains the information to recognize faces.
# Peruse the picture
picture = cv2.imread(imagePath)
dark = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
Here we read the picture and convert it to grayscale. Numerous activities in OpenCV are done in grayscale.
TRENDING - learn bash scripting
# Identify faces in the picture
faces = faceCascade.detectMultiScale(
dark,
scaleFactor=1.1,
minNeighbors=5,
minSize=(30, 30),
banners = cv2.cv.CV_HAAR_SCALE_IMAGE
)
This capacity identifies the genuine face and is the vital piece of our code, so we should go over the choices:
The detectMultiScale work is a general capacity that distinguishes objects. Since we are calling it on the face overflow, that is the very thing it identifies.
The main choice is the grayscale picture.
The second is the scaleFactor. Since certain countenances might be nearer to the camera, they would seem greater than the appearances toward the back. The scale factor makes up for this.
The recognition calculation utilizes a moving window to identify objects. minNeighbors characterizes the number of articles that are identified close to the ongoing one preceding it pronounces the face found. minSize, in the interim, gives the size of every window.
NOTE - Python for beginners
Note: I took usually involved values for these fields. In actuality, you would try different things with various qualities for the window size, scale factor, etc until you found one that turns out best for you.
The capacity returns a rundown of square shapes in which it accepts it tracked down a face. Then, we will circle over where it thinks it tracked down something.
print "Found {0} faces!".format(len(faces))
# Draw a square shape around the countenances
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (0, 255, 0), 2)
This capacity returns 4 qualities: the x and y area of the square shape, and the square shape's width and level (w , h).
We utilize these qualities to draw a square shape utilizing the underlying square shape() work.
cv2.imshow("Faces found", picture)
cv2.waitKey(0)
Eventually, we show the picture and trust that the client will press a key.
Eliminate advertisements
Really taking a look at the Results
How about we test against the ABBA photograph:
$ python face_detect.py abba.png haarcascade_frontalface_default.xml
Python face recognition model 1: Abba
That worked. What about another photograph:
Python face recognition model 2: wrong
That … isn't a face. Once more, we should attempt. I changed the boundaries and tracked down that setting the scaleFactor to 1.2 disposed of some unacceptable face.
Python face location model 2: fixed
What was the deal? Python for beginners
Indeed, the main photograph was taken genuinely close up with a top notch camera. The subsequent one appears to have been taken from a far distance and perhaps with a cell phone. To this end the scaleFactor must be altered. As I said, you'll need to set up the calculation dependent upon the situation to stay away from misleading up-sides.
Be cautioned however that since this depends on AI, the outcomes won't ever be 100 percent exact. You will improve an adequate number of results as a rule, however sometimes the calculation will recognize wrong items as countenances.
The last code can be seen as here. - Python for beginners
Reaching out to a Webcam
Imagine a scenario where you need to utilize a webcam. OpenCV gets each edge from the webcam, and you can then distinguish faces by handling each casing. You will require a strong PC, yet my five-year-old PC appears to adapt fine, as long as I don't move around something over the top.
Comments